What is a Selection Sort Algorithm? (Python)
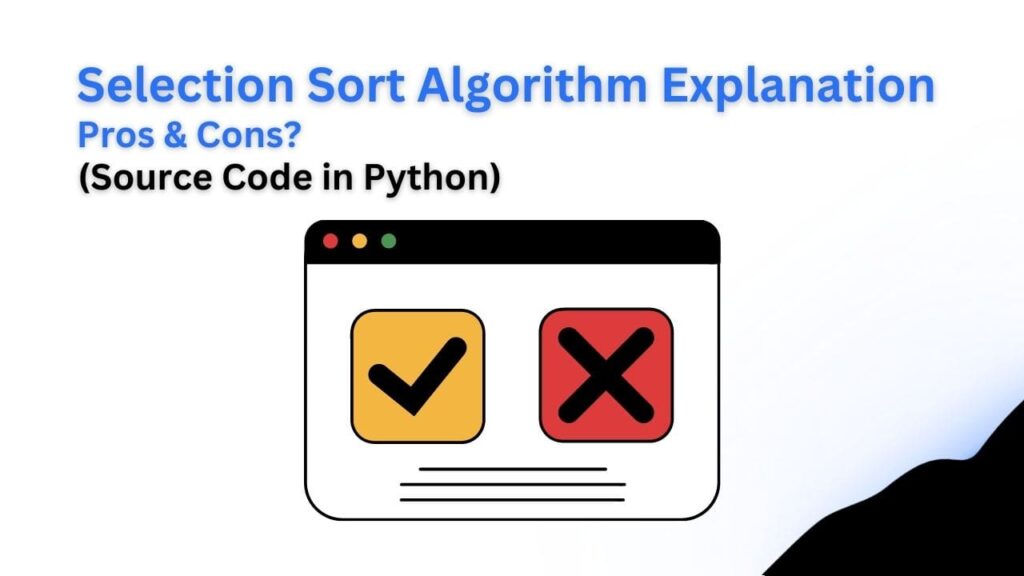
In this article, you will understand in detail how the Selection Sort Sorting Algorithm works as well as its implementation, advantages, and disadvantages. Source Code will be also provided.
What is Selection Sort Algorithm?
Selection Sort is the simplest sorting algorithm with a complexity time of O(n2). Selection Sort sorts the unsorted lists by constantly comparing the values to find the minimum to sort in ascending order.
Primarily, Selection Sort Algorithms have two main sub-lists, one of them is sorted and the other one is the unsorted list. An important rule to remember is that it doesn’t only output the sorted list, it swaps the numbers.
We’ll learn the concept of the Selection Sort Algorithm in depth below.
How does Selection Sort Algorithm work?
First of all, we need to understand that we would work with the two different values in the process of sorting the elements. These two values would be the Current Item and Current Minimum.
Let’s dive into it to understand how the selection sort algorithm works. Firstly, we will define the list that we are going to sort:
Number = [5,4,2,1,8]
And, our Current Item and Current Minimum are going to be always the first element while starting the selection sort.
(Step 1)
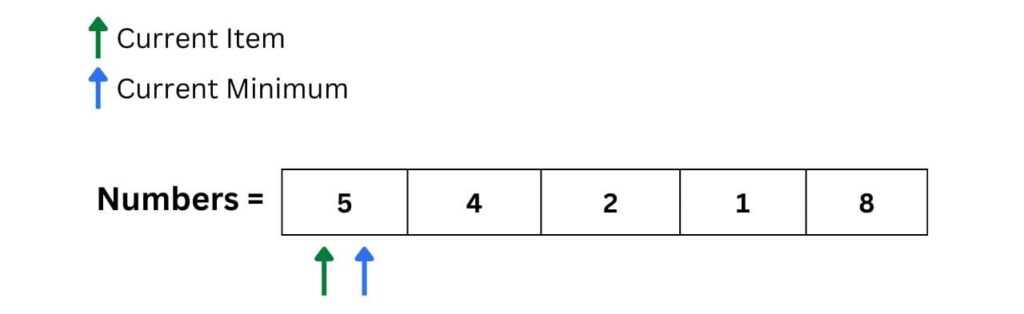
Now, we will try to find the next minimum number through the list, we will do that by constantly changing the Current Item and Current Minimum like this:
(Step 2)

(Step 3)

(Step 4)

(Step 5)

(Step 6)

(Step 7)

(Step 8)
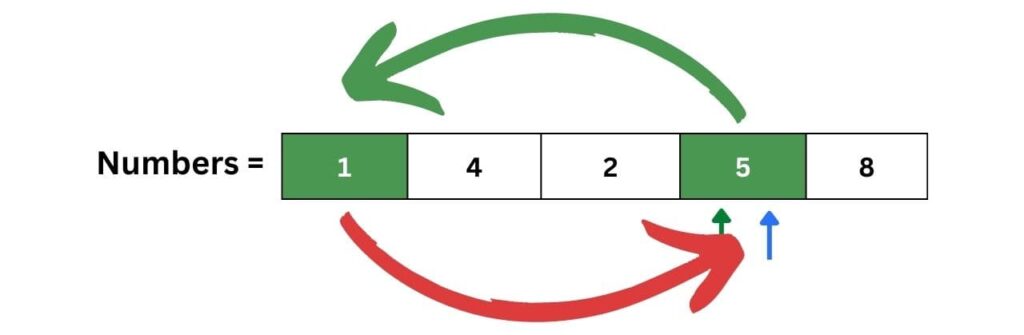
Notice that the sorting algorithm has compared the first element with the next element. Once it found the next element was smaller than the first one, it moved its Current Item and Current Minimum. This process is repeated till the sorting algorithm finds the smallest element in the list. Then, it swaps the elements. The same process goes over and over again to sort the list.
Another important point to remember here is that as it swaps the elements, it is creating two lists one of them is sorted and the other unsorted.
Ultimately, we have a list that looks like this:

What are the Advantages and Disadvantages of Selection Sort Algorithms?
Advantages of Selection Sort Algorithm:
★Doesn’t require more space: Selection Sort Algorithm requires only one variable space that will have a temporary minimum. Otherwise, it works around existing variables.
★Performs Well on Small Lists: Selection Sort is known for its great performance when working with small lists.
★Quite Easy to Implement: The Selection Sort Algorithm process is quite easy to understand and implement through the code.
Disadvantages of Selection Sort Algorithm:
★Inefficient for Large lists: Selection Sort Algorithm is very slow as compared to other sorting algorithms.
★Not Scalable: The fact that Selection Sort Algorithm isn’t efficient with large lists, it can’t be scaled from sorting small lists to large lists.
Python Source Code
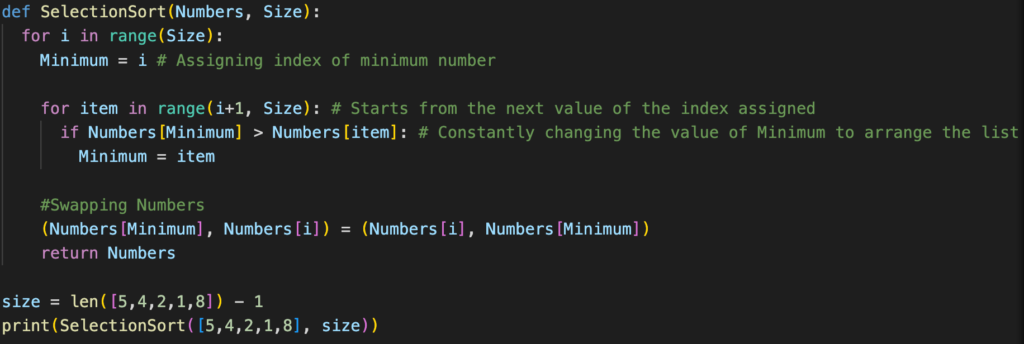
Conclusion
There are multiple advantages and disadvantages of Selection Sort Algorithms. Whilst it is the one of the simplest algorithms to implement, it is also quite slow.
I hope that you got value from this article if you would like to read other related articles. They will be mentioned below.
Good Luck!